## Going Isomorphic with React
[@bensmithett](https://twitter.com/bensmithett)
### Isomorphic JavaScript
Shared JavaScript that runs on both the client & server
### "Holy Grail" web app
- On first page load, serve real server-rendered HTML
- Client-side JS app bootstraps on top of server-rendered HTML
- rather than bootstrapping into an empty div
- From that point on, it's a client-side JS app
## [React](http://facebook.github.io/react/)
A JavaScript library for building user interfaces
The V in MVC
This is a React component:
```
var HiButton = React.createClass({
handleClick: function () {
alert("hi " + this.props.name);
},
render: function () {
return (
<button onClick={this.handleClick}>
Say hi
</button>
);
}
});
```
React.renderComponent(<HiButton name="Ben" />, document.body);
```
React.renderComponentToString(<HiButton name="Ben" />);
```
returns this string...
```
<button data-reactid=".1" data-react-checksum="762252829">
Say hi
</button>
```
React component + ability to run JS
=
Ability to render a React component to a HTML string
We can run JS on servers now...
... so we can render React components on the server!
### Let's talk about Views
### Let's talk about server-rendered Views
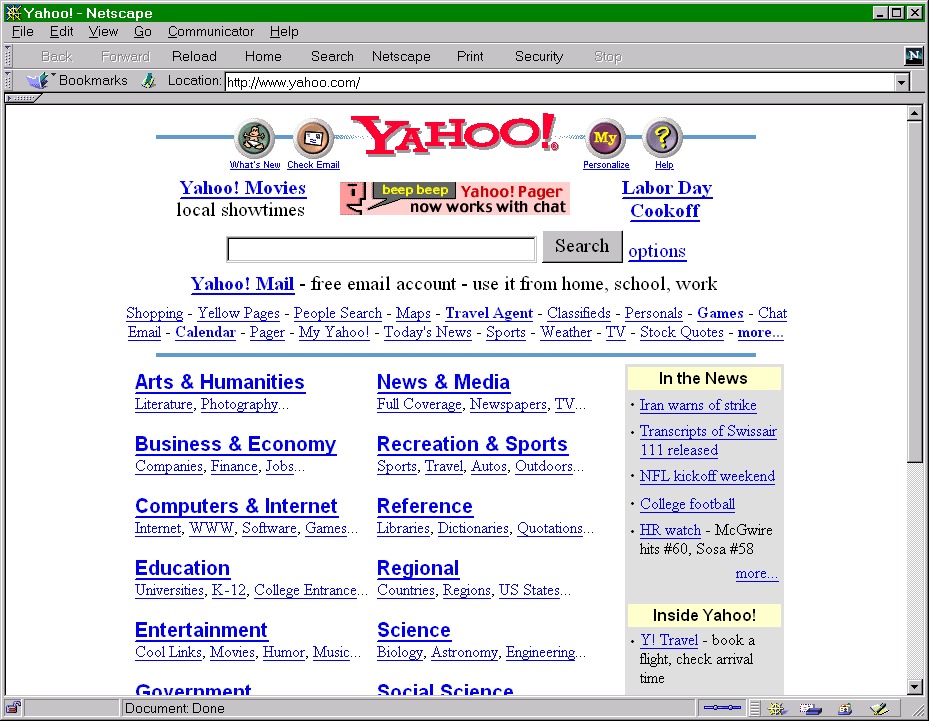
React is a pretty great option for server-rendered views.
Client-rendered view (Backbone)
Handlebars template
|
|
Server-rendered view (Rails)
ERB template
ERB template:
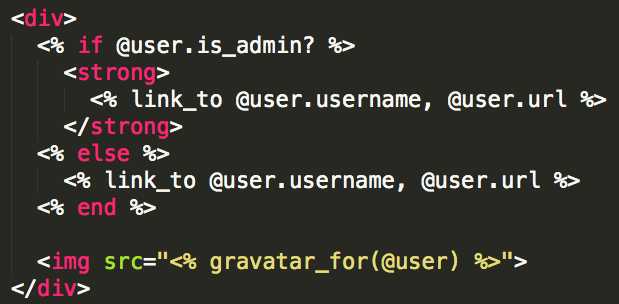
We give a template some data
It gives us back a string
Templates are just functions.
Early on they were big, ugly, complicated functions:
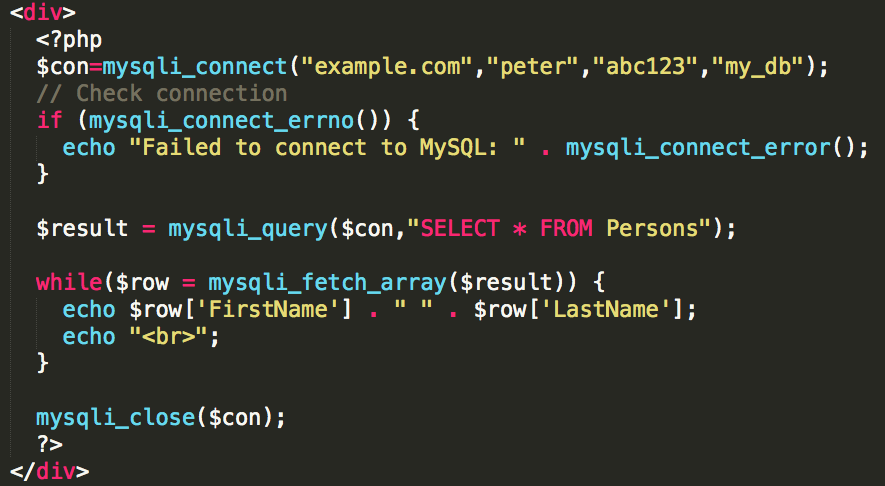
*Source: W3Schools*
We reacted to unmaintainable spaghetti templates
### "no logic in templates!"
But we wanted a little bit of flexibility...
### "no business logic in templates!"
(but the occasional loop or conditional is ok...)
Sometimes we need to do string manipulation, or sorting...
### "view logic belongs in ViewModels, not templates!"
(except loops & conditionals, which are still arbitrarily allowed in templates)
**Current state of play for server-rendered views:**
- *Mostly* logic-less template, but loops & conditionals are allowed
- Template logic written in the same language as other server code
- ViewModel/Presenter handles more complex logic
- Different files
A better server-rendered View: **React components**
#### So using React for server-side templates is awesome
#### Meanwhile, back in 2014...
client-side JS is a bit of a thing
Client-rendered view
Handlebars template
|
|
Server-rendered view
ERB template
Client-rendered view
React component
|
|
Server-rendered view
React component
## Isomorphic JavaScript!
Server
```
<body>
<%= React.renderComponentToString(<UserProfileView />); %>
</body>
```
Browser
```
React.renderComponent(<UserProfileView />, document.body);
```
When browser-React encounters HTML rendered by server-React, it just adds event listeners.
Same component file used on client & server!
But what if my back end isn't node.js?
**How can I do this in Rails/PHP/.NET/whatever?**
### View rendering as a service
Your app might already talks to other services over HTTP
- Search
- Authentication
- Third party APIs
*https://github.com/facebook/react/tree/master/examples/server-rendering*
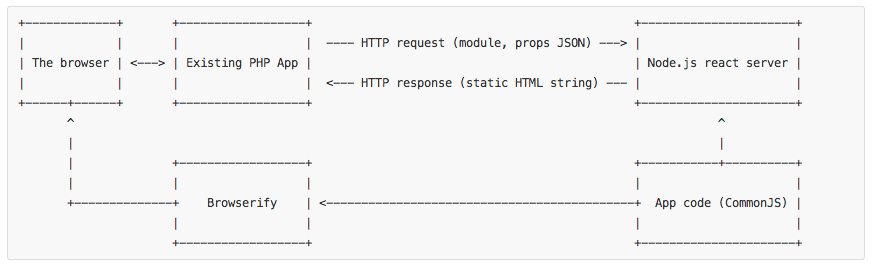
https://github.com/reactjs/react-rails
#### Bonus! Free progressive enhancement!
- You can render a view on the server based on application state at any point in time.
- If something goes wrong in the browser, no worries! Submit a form, click a link...
- The server will render the app in exactly the same state as the client JS would have.
# Thanks!
[@bensmithett](https://twitter.com/bensmithett)
-----
We're hiring awesome developers!
[envato.com/careers](http://www.envato.com/careers)
-----
[Server-rendered React components in Rails](http://bensmithett.com/server-rendered-react-components-in-rails/)
[React server-rendering examples](https://github.com/facebook/react/tree/master/examples/server-rendering)
[Airbnb post introducing Rendr](http://nerds.airbnb.com/weve-launched-our-first-nodejs-app-to-product/)